Introduction to Front-End Coding: A Beginner's Guide
Front-end development involves using HTML, CSS, and JavaScript to create the visual and interactive aspects of websites, allowing users to interact with them directly. Through a simple experiment of building a basic web page, beginners can start to understand these core technologies, setting the foundation for further learning and exploration in web development.
INFORMATIVECODE
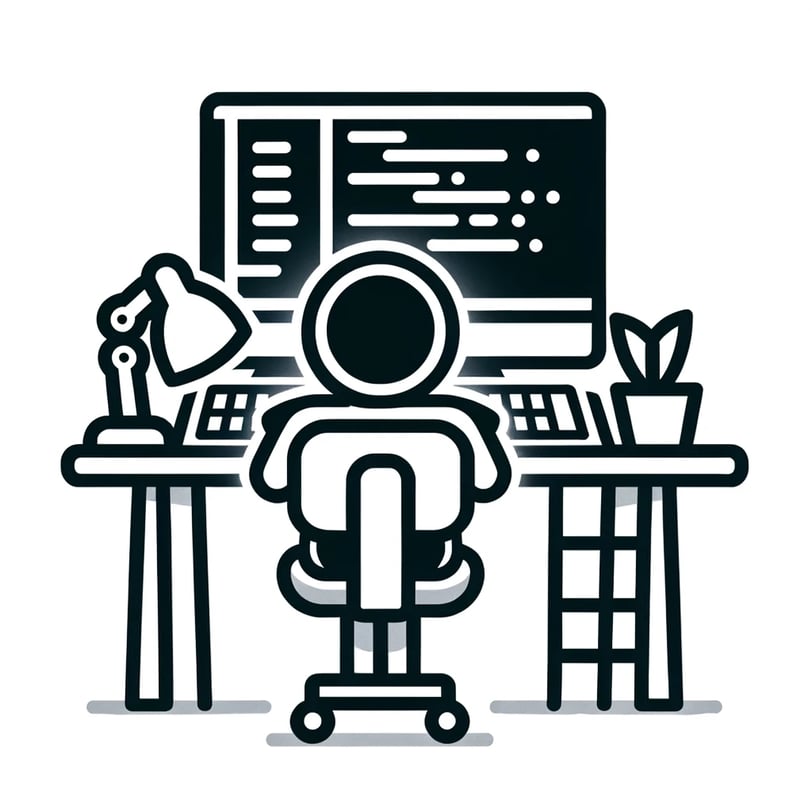
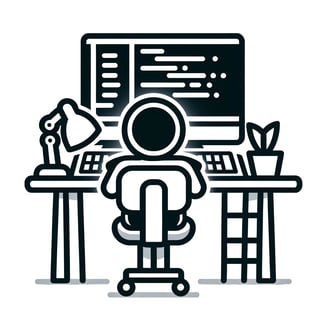
Welcome to the fascinating world of front-end development, where the visual aspects of websites come to life. Front-end coding, also known as client-side development, involves creating the graphical user interface (GUI) through which users interact with websites in their web browsers. This guide aims to introduce you to the basics of front-end development and provide a hands-on experiment to kickstart your journey.
Understanding Front-End Development
Front-end development primarily uses three core languages: HTML (HyperText Markup Language), CSS (Cascading Style Sheets), and JavaScript. Each plays a distinct role in website creation:
- HTML provides the basic structure of sites, which is enhanced and modified by other technologies like CSS and JavaScript.
- CSS is used to control presentation, formatting, and layout.
- JavaScript is used to control the behavior of different elements.
Together, these technologies allow developers to create rich, interactive web experiences.
The Role of Front-End Developers
Front-end developers focus on the user interface and user experience. They take the design and transform it into a functional website. They ensure that the website is responsive, accessible, and performs well across different devices and browsers.
Practical Experiment: Building a Simple Web Page
To give you a taste of front-end development, let's create a simple web page that introduces you to HTML and CSS.
What You'll Need
- A text editor (Notepad, Sublime Text, Visual Studio Code, etc.)
- A web browser (Chrome, Firefox, Safari, etc.)
Step 1: Setting Up Your HTML Document
Create a new file in your text editor and save it as `index.html`. Then, type the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First Web Page</title>
</head>
<body>
<h1>Welcome to Front-End Coding!</h1>
<p>This is my first attempt at creating a web page using HTML and CSS.</p>
<button id="clickMe">Click Me!</button>
</body>
</html>
This code defines a basic structure of a web page. Most tags (<>) have closing tags (</>) unless self-closing. The `<h1>` tag creates a large heading, and the `<p>` tag creates a paragraph. The `<button>` tag creates a clickable button.
Step 2: Adding Some Style with CSS
Now, let's add some style to our page. Inside the `<head>` section of your HTML document, add the following `<style>` tag:
<style>
body {
font-family: Arial, sans-serif;
margin: 40px;
text-align: center;
}
button {
background-color: #4CAF50;
color: white;
padding: 15px 20px;
margin: 20px 0;
border: none;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
</style>
This CSS code changes the font of the text, centers the content, styles the button with a background color, and defines a hover effect. This is for ease of understanding, typically you would save this as a separate file, styles.css, and call that file from your html with <link rel="stylesheet" type="text/css" href="styles.css"> but both work the same.
Step 3: Adding Interactivity with JavaScript
Let's make the button interactive. Add the following `<script>` tag just before the closing `</body>` tag:
<script>
document.getElementById('clickMe').onclick = function() {
alert('You clicked the button!');
};
</script>
This JavaScript code displays an alert box when the button is clicked. As with the .css file, this should be its own script.js file ad called with <script src="script.js"></script> but is not a requirement.
Step 4: Viewing Your Web Page
Save your `index.html` file and open it in a web browser. You should see a styled web page with a heading, a paragraph, and a clickable button that shows an alert when clicked. If using seperate files for .css and .js, make sure they are in the same folder and level as the .html file.
Conclusion
Congratulations! You've just created your first web page using HTML, CSS, and JavaScript. This experiment is a simple demonstration of what front-end development entails. As you continue to learn and experiment, you'll discover the vast possibilities of creating interactive and dynamic web experiences.
Front-end coding is an exciting and creative field, constantly evolving with new technologies and practices. Start exploring more complex projects, experiment with different frameworks like React or Vue.js, and consider contributing to open-source projects to build your skills and portfolio.
Remember, the key to becoming proficient in front-end development is practice and continuous learning. What project will you tackle next to hone your newfound skills?